I keep a bunch of handy code fragments here so I don’t lose them. They are in no particular order.
IEnumManager – Get IState, IBillCode, and Many Others
You must use IEnumManager to get literally dozens of values that are come from lists that are either maintained inside Select (like IState) or are user editable (such as Bill Code values in the SPAdmin Drop-down Lists). The technique is the same.
from SoftPro.Select.Client.Enumerations import * # Set address state to North Carolina. enumMgr = order.GetService(IEnumManager) states = enumMgr.GetEnum[IState]() state = next(t for t in states.Values if t.Code == "NC") address.State = state # Works for Bill Codes too. enumMgr = order.GetService(IEnumManager) bcs = enumMgr.GetEnum[IBillCode]() bc = next(t for t in bcs.Values if t.Code == "END") charge.BillCode = bc
Using LINQ and IAccountsManager
Using LINQ and also using the IAccountsManager service require special handling in Select CORs or snippets.
from System import * from SoftPro.ClientModel import * from SoftPro.OrderTracking.Client import * from SoftPro.OrderTracking.Client.Orders import * # Add LINQ support. import clr import System clr.AddReference('System.Core') clr.ImportExtensions(System.Linq) # Must load "SoftPro.Accounting.Client.dll" before referring to it. # Needed for "IAccountsManager" service. clr.AddReference('SoftPro.Accounting.Client') from SoftPro.Accounting.Client.Accounts import * # Fill "Project name" field with Guid for trust account specified in "Email subject line" def Order_Project_Value(args): acctMgr = args.Context.GetService(IAccountsManager) ta = (IAccountsManager.TrustAccounts.GetValue(acctMgr) .Where(lambda t: t.Code == args.Context.EmailSubjectLine and t.Enabled == True) .FirstOrDefault()) if ta is None: args.Value = "Not valid or disabled trust account." else: # Print Guid. args.Value = '--> ' + str(ta.ID)
Once a valid and non-disabled trust account Code is added to the “Email subject line” field, the “Project name” displays the guid.
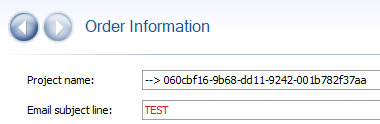
Out/Ref Parameters – clr.Reference[T]
This is an example where we convert a string to a Guid. It requires an out parameter. It boils down to creating a reference variable then using the value by using the “Value” property of the reference.
# Convert string to guid. If invalid, exit COR. sin = '060cbf16-9b68-dd11-9242-001b782f37aa' gout = clr.Reference[System.Guid]() # TryParse will return True if it can convert the string to the output type (gout). # Otherwise will return False and "gout" is unset. if (not Guid.TryParse(sin, gout)): # Bad guid string format. ... else: # Use value. print str(gout.Value)